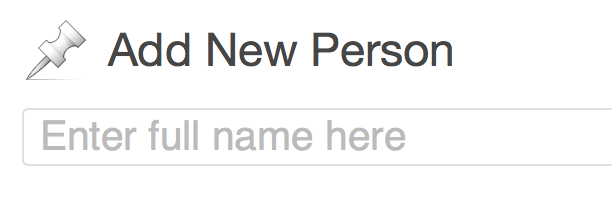
On fazd.tamu.edu, we found that in addition to the standard pages and posts, we had three distinct things: research projects, tools (the tangible items created during past research projects, like software suites or new vaccines), and people. These will become our custom post types.
For the research projects, we need to display a few things as distinct fields: the start and end dates of the grant, and the amount of money awarded for the research.
The tools don’t have dates or money attached anymore, but there is a photo or screenshot we can use to represent each one. We don’t need a custom field for that, though — the built-in featured image will be fine. We just need to enable featured images in the theme.
For people, we’ll use the post title field to store the full name, with appended degrees, as it will be displayed in most situations. (I’ll tweak WordPress’s built-in prompt to help the site’s content authors get this right; I’ll talk more about that in a minute.) However, we do need to alphabetize lists of people, so we need to break out their last names into a separate custom field. Their contact information (phone, email, URL, maybe a Twitter handle) should also go into separate fields so we can pull those into a staff directory. The post body will do just fine for the person’s bio, and and the featured image can store their portrait.
Here’s a diagram of the complete content model:
The items in yellow (projects, tools, people) are the post types. The custom fields are listed beneath each post type’s name. The big curved arrows between the post types represent the Posts2Posts connections. The smaller arrows represent the taxonomies’s relationships to the custom post types. Each taxonomy (shown here as a white checkbox list) is shared by at least two post types. Institutions is a taxonomy shared by all the post types: for tools and research projects, it represents the research groups that have partnered on the project. People are listed under each institution for which they work — there might be more than one, in cases of dual academic appointments.
This diagram is slightly simplified — the site actually has two more taxonomies and another (unrelated) custom post type.
So here’s what we’re going to build over the next few posts in this series:
Custom post types:
- projects
- tools
- people
Custom taxonomies:
- institutions (for projects, tools, and people)
- topics (for projects and tools)
Custom field groups:
- Research project information (for projects)
- Start date
- End date
- Award amount
- Name and job title (for people)
- Last name
- Job title
- Contact information (for people)
- Phone number
- Email address
- URL
- Twitter handle
Tweaking WordPress’s Prompts
Consider what a “people” post type (hereafter referred to as a person) will look like in the Dashboard. It’ll be a regular post with a title and body field. We plan to use the post title for the full name. However, there’s also a custom field that will contain a job title. That could be confusing for someone who doesn’t use WordPress on a regular basis, especially because the default placeholder text in the post title box says “Enter title here.”
We can change that!
In “Mangling Strings for Fun and Profit,” core developer Peter Westwood shows us how to change WordPress’s built-in text by taking advantage of its translation features. Bad news: it does require you to write some code. Good news: you can just cut and paste from Peter’s example, replacing the old and new phrases with the ones you want.
I’m skipping ahead a little bit here, so for now just know that we’re going to use “people” as the name of our custom post type for people. And here’s how we would change the title field’s placeholder/prompt on the people editing page, but not regular posts or pages:
// Change placeholder text on edit/add Person page class FAZD_Translation_Mangler { function filter_gettext($translation, $text, $domain) { global $post; $translations = &get_translations_for_domain( $domain ); if ( $post->post_type == 'people' && $text == 'Enter title here' ) { return $translations->translate( 'Enter full name here' ); } return $translation; } } add_filter('gettext', array('FAZD_Translation_Mangler', 'filter_gettext'), 10, 4);
Here’s the result:
There are lots of little things like this that you can tweak in order to clarify or simplify the editing screens for your custom post types.
In the next post, I’ll show you the code you need to start building the post types!
If you want all the code right this minute, grab my new book, WordPress for Web Developers. The final chapter walks you through the complete process of building custom post types, taxonomies, and fields.
Wow. I really like that string mangler. In the past I’ve always removed title support for the CPT and saved one of the metaboxes as the title using the ‘title_save_pre’ filter. This solution will definitely come in handy.
Isn’t it nifty? You do have to be careful with it. I once forgot to return a value, and all the month names disappeared from the dates. (I guess those were the only things being translated.)
Thank you for the 3 parts. I also read chapter 4. So now it’s time for da book!